Solving the Dining Savages Problem: Synchronization Strategies
Written on
Chapter 1: The Dining Savages Challenge
The Dining Savages problem presents an intriguing synchronization puzzle where a group of savages must coordinate their communal meals effectively. The scenario involves a large pot that can hold a limited number of servings (M) for their dinner. When a savage wishes to eat, they will serve themselves from the pot unless it is empty. If the pot is depleted, the savage must wake the cook, who is solely responsible for replenishing the pot.
To illustrate this scenario programmatically, we can visualize each savage and the cook as distinct threads executing specific operations. The cook's primary duty is to refill the pot when it runs out of food. Savages, on the other hand, continually attempt to serve themselves. If the pot is empty, they must signal the cook to refill it before they can eat.
Below is the unsynchronized pseudo-code depicting the actions of the cook and savages:
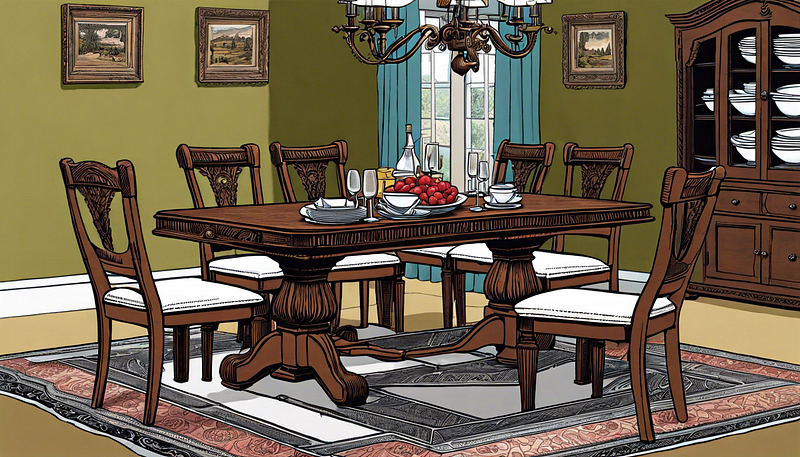
The unsynchronized pseudo-code above reveals a critical flaw: the cook cannot execute putServicesInPot() when the pot has contents, and savages cannot call getServingFromThePot() if the pot is empty. The goal is to implement synchronization mechanisms that ensure both conditions are met.
Chapter 2: Synchronization Strategies
To address the synchronization challenge, we can consider several hints:
The video titled "OS34 - Dining Philosophers Problem with Semaphores" provides valuable insights into solving synchronization issues in similar scenarios.
Hint 1: One potential approach is to utilize a semaphore to monitor the number of servings in the pot. However, this may not be effective because savages need to assess the remaining servings and signal the cook accordingly, which is not feasible with a semaphore alone. Thus, using a simple integer variable to track the number of servings becomes the preferred option.
Hint 2: We must also ensure that the cook only invokes putServingsInPot() when the pot is empty, triggered by a savage's signal. A binary semaphore can serve this purpose, allowing savages to indicate when the pot is empty. Only one savage should signal the semaphore when the pot is devoid of servings.
Hint 3: Once the pot is replenished, the cook needs to notify the waiting savages. Another binary semaphore can facilitate this communication. After refilling the pot, the cook signals this semaphore, allowing the savage who prompted the refill to proceed.
Hint 4: Lastly, we need to guarantee that access to serving variables is thread-safe, ensuring that only one savage signals the cook when the pot is empty. A mutex can be utilized for this purpose, preventing simultaneous attempts by multiple savages to access the pot.
The following diagram summarizes the necessary variables for solving this problem:
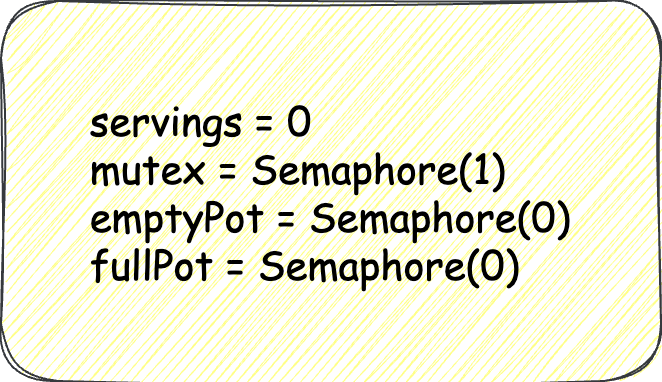
Chapter 3: Implementing the Solution
Building on the hints discussed, we can outline the synchronized code for the cook. The cook will wait for a signal indicating that the pot is empty. Upon receiving this signal, the cook will refill the pot with M servings and notify the savages once this is complete.
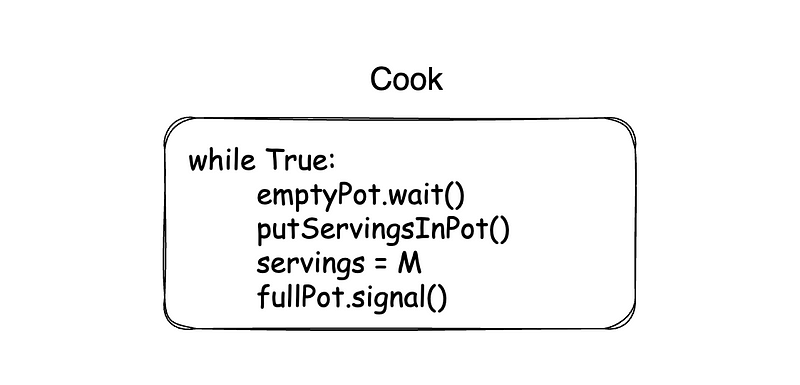
Now, let's examine the code for the savages. When they attempt to take a serving, they must first acquire the mutex. If servings are available, they will retrieve their portion and release the mutex. If the pot is empty when they try to serve, they will signal the cook to refill it and wait for the cook to complete this action.
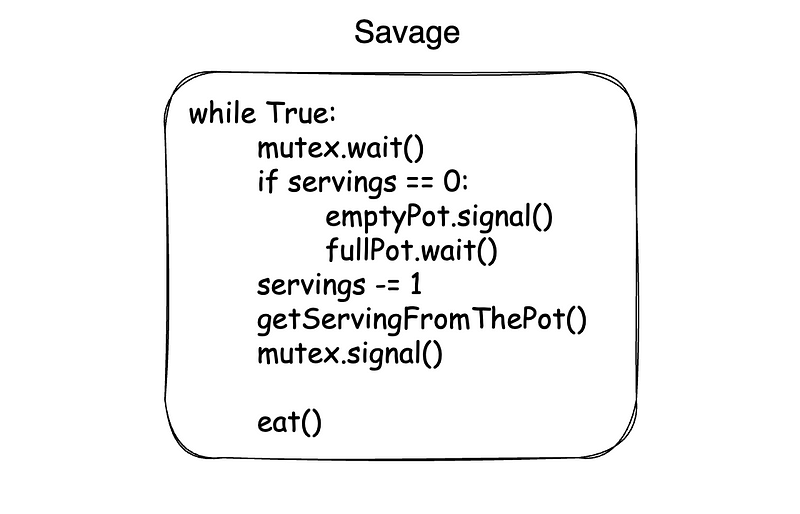