Understanding JavaScript For Loops and ForEach Method
Written on
Chapter 1: Introduction to For Loops and ForEach
When starting your journey with JavaScript, distinguishing between for loops and forEach can be quite challenging. At first glance, they appear to serve the same purpose: iterating over arrays or collections of data. However, understanding the fundamental differences is crucial for effective usage. In this article, we will clarify the distinctions between for loops and forEach and guide you on their proper application.
Syntax Comparison
The most noticeable difference lies in their syntax. A standard for loop is structured as follows:
for (let i = 0; i < array.length; i++) {
// Access each element with array[i]
}
This loop will continue executing as long as the condition (i is less than the length of the array) holds true. You can access each element of the array using the index variable āiā.
Conversely, the forEach method is written like this:
array.forEach((element) => {
// Perform operation on element
});
This syntax iterates through each item in the array, executing the specified operation. For many developers, the forEach syntax is more concise and easier to understand.
Performance Considerations
Another significant difference involves performance. Typically, for loops execute faster than forEach because they avoid the overhead associated with function calls for each element. However, this performance gap is often minimal unless dealing with exceptionally large arrays.
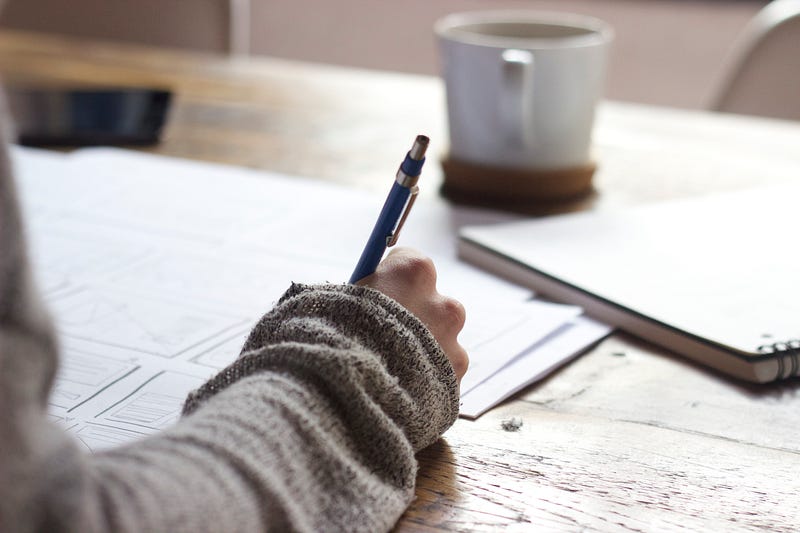
Caveats of Using forEach
One limitation of the forEach method is that it does not allow you to exit the loop prematurely. If you find yourself needing to break out of the loop before it reaches its conclusion, a traditional for loop would be necessary.
Additionally, forEach does not return values. For instance, if your goal is to iterate through an array to find the first element starting with the letter āAā, forEach would not suffice. In such cases, you would need to revert to a standard for loop.
Moreover, it's important to note that the forEach method may produce unexpected results when combined with the await keyword. It was not designed to handle asynchronous functions, meaning that using an async function within forEach could lead to unpredictable execution order.
Conclusion
While the forEach method is generally more readable and can leverage arrow functions for succinctness, it has its drawbacks. Recognizing when to apply forEach versus a traditional for loop is essential for effective coding practices.
We hope this article has clarified any uncertainties regarding these JavaScript features. Best of luck with your coding endeavors!