Creating Graphical User Interfaces in Python with PySimpleGUI
Written on
Chapter 1: Introduction to GUI Development
Developing graphical user interfaces (GUIs) with Python has become remarkably straightforward. Utilizing the PySimpleGUI module, you can effortlessly design and construct GUIs while enjoying a high degree of customization.
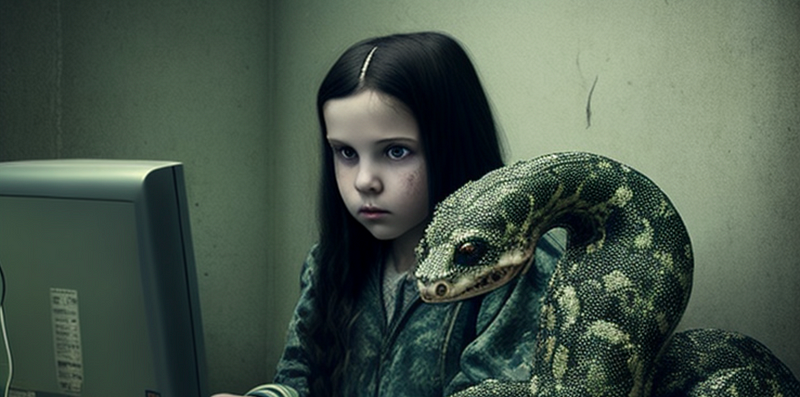
In this guide, I'll walk you through the steps to create a GUI application using Python along with PySimpleGUI. We will explore the fundamentals of the module, including installation, creation of essential components like buttons and text fields, and how to manage events and user inputs. By the end of this guide, you’ll have a firm grasp of how to build GUI applications with Python and PySimpleGUI, equipping you to embark on your own projects. Let’s dive in!
One of the key benefits of PySimpleGUI is its user-friendly nature. This module is crafted for ease of use, making it suitable for novices in Python or GUI development. Its syntax is clear and intuitive, allowing for the quick creation of basic elements like buttons and text fields. Moreover, PySimpleGUI offers an extensive array of widgets, enabling the construction of sophisticated, feature-rich applications without delving into the complexities of GUI programming.
Another significant advantage of PySimpleGUI is its adaptability. The module works seamlessly across various platforms, including Windows, Mac, and Linux, and it supports both Python 2 and Python 3. This compatibility means you can develop applications that function on nearly any computer. Additionally, PySimpleGUI is highly customizable, allowing you to modify your application to suit your specific requirements and preferences.
PySimpleGUI is also recognized for its robustness and efficiency. Built on the Tkinter library—an established and powerful library for GUI programming in Python—PySimpleGUI unlocks a wealth of advanced features and functionalities that might be unexpected from such a straightforward library. Plus, it is lightweight, ensuring that your applications operate swiftly and efficiently.
In conclusion, PySimpleGUI stands out as an exceptional choice for creating GUI applications with Python. Its simplicity, flexibility, and power make it ideal for both beginners and seasoned developers. Whether you aim to create a basic application or a more intricate, feature-rich program, PySimpleGUI provides everything you need to get started. If you're ready to build GUI applications with Python, explore what PySimpleGUI has to offer and see how it can streamline your development process.
Chapter 2: Getting Started with PySimpleGUI
Now that we’ve discussed the advantages of PySimpleGUI, let's explore how to get started with it. The initial step is to install the module, which can be done by executing the following command in your command prompt or terminal:
pip install pysimplegui
After installing PySimpleGUI, you can begin constructing your GUI application. The fundamental building block of a PySimpleGUI application is the window. A window serves as the primary container for all your widgets, including buttons and text fields. Below is a simple example of how to create a basic window:
import PySimpleGUI as sg
layout = [[sg.Text('Hello World')],
[sg.Button('Ok')]]
window = sg.Window('My First Window', layout)
while True:
event, values = window.read()
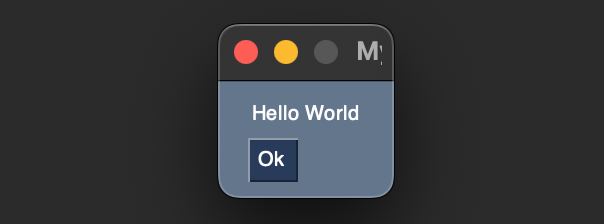
In this snippet, we first import the PySimpleGUI module and assign it the alias “sg.” Next, we define a layout for our window as a nested list of elements, where each element symbolizes a widget. In this instance, we include a text element that displays “Hello World” and a button labeled “Ok.” Finally, we create a window titled “My First Window” with the specified layout.
You can also design more intricate layouts incorporating various widgets like input text boxes, dropdowns, radio buttons, and checkboxes. For example, here’s a more advanced layout featuring a text box for entering a name, a dropdown for selecting a color, and a submit button:
import PySimpleGUI as sg
layout = [[sg.Text('Enter your name:'), sg.InputText()],
[sg.Text('Select your favorite color:'), sg.Combo(['Red', 'Green', 'Blue'], size=(20, 1))],
[sg.Submit()]]
The window creation follows the same procedure as before. Once you’ve prepared your layout, it’s essential to run an event loop to manage user interactions with your GUI. The event loop will persist until the user closes the window or clicks a button that instructs it to exit:
while True:
event, values = window.read()
if event in (None, 'Submit'): # if user closes the window or clicks submit button
breakprint(f'The entered name is {values[0]} and the selected color is {values[1]}')
In this case, we maintain a while loop that continues until the user either closes the window or presses the submit button. The window.read() method returns an event and values, reflecting the user’s actions and any entered data. We can evaluate the event to determine the user’s actions and respond accordingly. In this example, we print the entered name and selected color to the console.
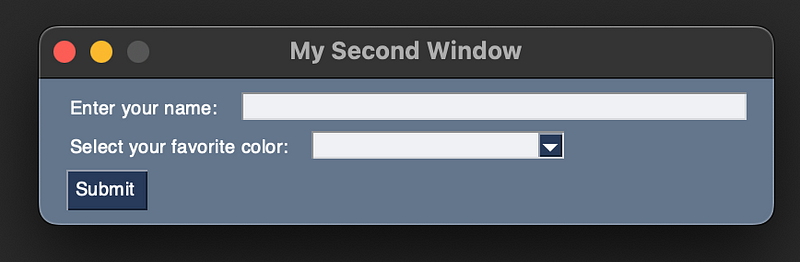
With these foundational concepts, you can create a diverse array of GUI applications using PySimpleGUI. You may delve into additional widgets, layout possibilities, and customization features to design more complex and feature-rich applications. By leveraging PySimpleGUI, you can effortlessly craft user-friendly and visually engaging interfaces for your Python applications, enhancing their accessibility and appeal.
Chapter 3: Advanced Techniques in PySimpleGUI
With a basic understanding of PySimpleGUI under your belt, let's examine some advanced techniques for crafting even more dynamic and flexible GUI applications.
One of PySimpleGUI's most impressive capabilities is its support for multiple windows. This functionality allows you to develop complex applications featuring several screens, each equipped with its own controls and functionalities. Here is an example illustrating how to create and toggle between two windows in your application:
import PySimpleGUI as sg
layout1 = [[sg.Text('This is window 1')], [sg.Button('Open Window 2')]]
layout2 = [[sg.Text('This is window 2')], [sg.Button('Close')]]
window1 = sg.Window('Window 1', layout1)
window2 = sg.Window('Window 2', layout2)
while True:
event, values = window1.read()
if event == 'Open Window 2':
window1.hide()
event, values = window2.read()
if event == 'Close':
window2.hide()
window1.un_hide()
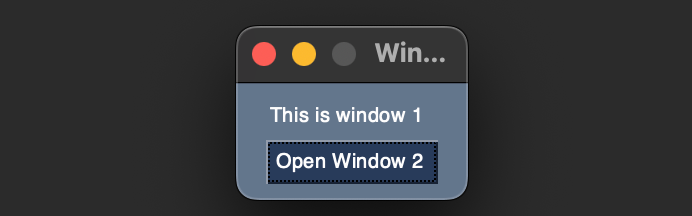
This example generates two windows: "Window 1" and "Window 2," each with its unique layout and controls. The script operates an event loop that monitors user actions and values, switching between the two windows when the button in the first window is pressed and closing the second window when its button is clicked.
Another advanced technique you can implement in PySimpleGUI is multi-threading. This allows for the concurrent execution of multiple tasks, significantly enhancing the performance and responsiveness of your application. Below is an example showcasing how to utilize multi-threading in PySimpleGUI:
import PySimpleGUI as sg
import threading
import time
def some_long_task():
for i in range(5):
print(f'Running task {i}')
time.sleep(1)
layout = [[sg.Text('Press the button to start the task')],
[sg.Button('Start Task'), sg.Button('Cancel')]]
window = sg.Window('My Task', layout)
while True:
event, values = window.read()
if event == 'Start Task':
threading.Thread(target=some_long_task).start()elif event == 'Cancel':
break
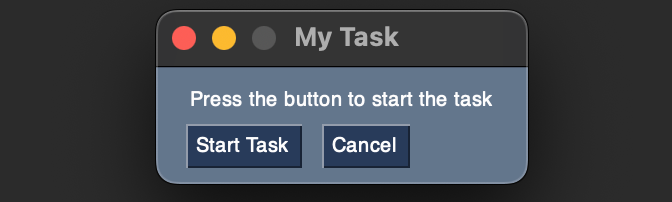
This script constructs a window with two buttons: one for starting a task and another for canceling it. When the user clicks the “Start Task” button, a new thread is initiated that runs the some_long_task function, simulating a lengthy task. This design allows the main thread to continue executing the event loop and keeps the GUI responsive. The user can also press the "Cancel" button to halt the task and close the window.
In summary, PySimpleGUI is a robust and versatile module that simplifies the creation of GUI applications with Python. Its user-friendly yet powerful design allows you to create visually appealing and intuitive interfaces for your applications. With advanced features like multiple windows and multi-threading, you can develop even more powerful and responsive applications. By mastering these advanced techniques, you can elevate your PySimpleGUI skills and create truly exceptional GUI applications with Python.
I trust this guide has equipped you with valuable insights into utilizing PySimpleGUI to build potent and flexible GUI applications with Python. With this powerful module, your options are limitless, enabling you to develop visually engaging and user-friendly interfaces for a wide array of applications.
If you’re eager to expand your knowledge on PySimpleGUI and other topics in Python development, be sure to subscribe to my channel and join my newsletter. You’ll gain access to exclusive content, tutorials, and tips designed to enhance your Python programming skills.
For further resources, tutorials, and examples, check out the official PySimpleGUI website—it’s an excellent starting point. With the knowledge and skills you've acquired from this guide, you're well on your way to becoming a PySimpleGUI expert, ready to create outstanding GUI applications with Python. Don't hesitate to explore, experiment, and enjoy the process!
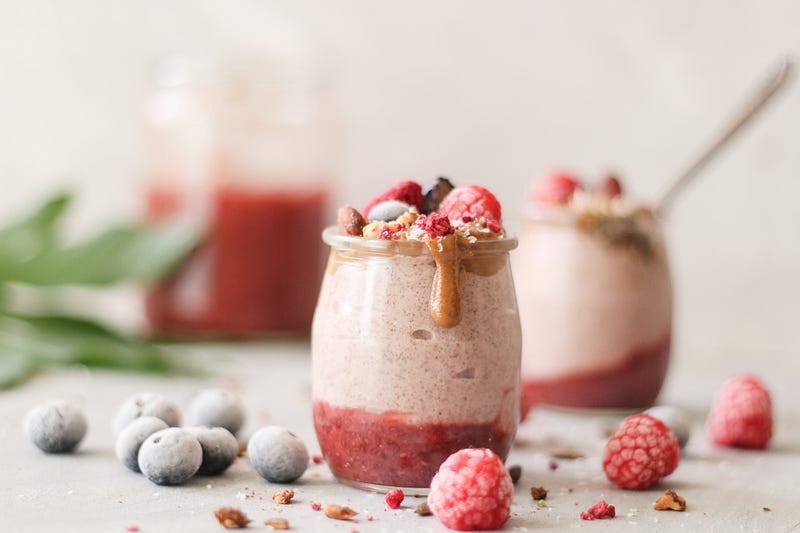
A comprehensive tutorial on creating advanced GUI applications with PySimpleGUI.
An ultimate introduction to PySimpleGUI, guiding you through the process of creating apps in Python.