Creating a Dynamic Flashcard Application Using React and JavaScript
Written on
Chapter 1: Introduction to React and Flashcards
In this guide, we will explore how to develop a flashcard application utilizing React alongside JavaScript. React is a user-friendly JavaScript framework designed for creating front-end applications.
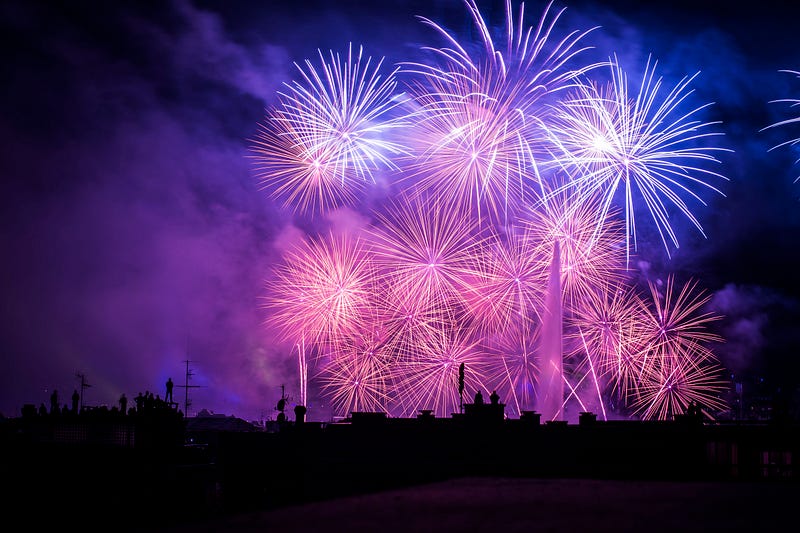
Photo by ben o'bro on Unsplash
Chapter 2: Setting Up the Project
To initiate our React project, we can leverage the Create React App tool. The installation command is as follows:
npx create-react-app flashcard
Next, we will require the uuid package to generate unique identifiers for our flashcard entries. To install it, simply execute:
npm i uuidv4
Chapter 3: Developing the Flashcard Application
To construct the flashcard application, we will need to implement the following code:
import { useState } from "react";
import { v4 as uuidv4 } from "uuid";
export default function App() {
const [item, setItem] = useState({});
const [items, setItems] = useState([]);
const add = (e) => {
e.preventDefault();
const { question, answer } = item;
const formValid = question && answer;
if (!formValid) {
return;}
setItems((items) => [
...items,
{
id: uuidv4(),
...item
}
]);
};
const deleteItem = (index) => {
setItems((items) => items.filter((_, i) => i !== index));};
return (
<div className="App">
<form onSubmit={add}>
<div>
<label>Question</label>
<input
value={item.question}
onChange={(e) =>
setItem((item) => ({ ...item, question: e.target.value }))}
/>
</div>
<div>
<label>Answer</label>
<input
value={item.answer}
onChange={(e) =>
setItem((item) => ({ ...item, answer: e.target.value }))}
/>
</div>
<button type="submit">Submit</button>
</form>
{items.map((item, index) => {
return (
<div key={item.id}>
<b>Question</b>
<p>{item.question}</p>
<b>Answer</b>
<p>{item.answer}</p>
<button onClick={() => deleteItem(index)}>Delete</button>
</div>
);
})}
</div>
);
}
In this code snippet, we utilize the useState hook to manage our item and items state. The add function handles adding a new item to the list. By invoking e.preventDefault(), we prevent the default form submission behavior and check if both the question and answer fields are filled. If they are, we call setItems to append the new item to the existing list. The deleteItem function allows us to remove an entry by filtering it out based on its index.
The form is set to trigger the add function upon submission, and the input fields are bound to their respective state properties. Each item is rendered with a unique key, ensuring React can efficiently manage the list.
Chapter 4: Conclusion
Creating a flashcard application using React and JavaScript is straightforward and efficient. This guide has provided a comprehensive overview of the necessary steps and coding practices.
The first video, "How To Build A Flashcard Quiz With React," offers a visual guide on developing a flashcard quiz application.
The second video, "React Firebase Flashcards App Tutorial," provides insights into integrating Firebase with your flashcard application for enhanced functionality.